Create a Project
Let's create a project.
$ npm install -g ionic cordova
$ ionic start sharedComponentDemo
This will take a while to download the necessary node packages. After that, we can go ahead to add pages and components. We'll use hero form in the demo. We'll have two pages that use the same form. The first page is to add a hero and the second page is to edit the hero. It makes sense to share the same form component.
Add Pages
We'll use the Ionic command to add pages. We'll make them lazy loading later.
First, let's create the page to add a hero.
$ ionic g page hero.add
That will create three files:
$ ls src/pages/hero-add/ hero-add.html hero-add.scss hero-add.ts
Then let's create a page to edit a hero
$ ionic g page hero.edit
Wire Pages to App
If you run ionic serve
now, you won't see the page. That's because the new pages we just added aren't wired to the app. And the pages created from the command aren't lazy loadable either. We're going to make them lazy loadable and add them to the application. We'll only use HeroAddPage as an example. We can apply the same change to HeroEditPage.
First, we need to import IonicPage
from ionic-angular in hero-add.ts, and add the decorator @IonicPage
to the class HeroAddPage (above @Component
).
Then, we'll create a module.
import { NgModule } from '@angular/core';
import { IonicPageModule } from 'ionic-angular';
import { HeroAddPage } from './hero-add';
@NgModule({
entryComponents: [
HeroAddPage,
],
exports: [
HeroAddPage,
],
declarations: [
HeroAddPage,
],
imports: [
IonicPageModule.forChild(HeroAddPage),
],
providers: [
],
})
export class HeroAddModule {}
We have the module for the page now. We can use it in the application. The Ionic command creates the app using the tab template. Let's change tabs.ts. Replace tab1Root with "HeroAddPage"
and tab2Root with "HeroEditPage"
and remove other tabs. Now we show the pages in the app. We don't need to import the modules or components. We just use the pages in the form of string. Ionic will load them.
A Shared Component
The shared component is a form for adding or editing a hero. Let's still use the command to create it.
$ ionic g component hero.form
Similar to the pages, we create the component and the module. I'll omit the component since it's similar to page. It just doesn't use @IonicPage
. I'll focus on the module. We have to export the component.
import { NgModule } from '@angular/core';
import { FormsModule } from '@angular/forms';
import { IonicModule } from 'ionic-angular';
import { HeroFormComponent } from './hero-form';
@NgModule({
entryComponents: [
],
exports: [
HeroFormComponent,
],
declarations: [
HeroFormComponent,
],
imports: [
FormsModule,
IonicModule,
],
providers: [
],
})
export class HeroFormModule {}
That's it. We just create a reusable component that can be used in different modules. There is one more step though. We need to import it in the modules where we use it. For example, in hero-add.module.ts, we add HeroFormModule into imports
.
1. Add the component to a shared module and export the component in the module.
2. Import the shared module in the modules where we'll use the component.
You can check out the complete demo app at https://github.com/kceiw/sharedComponentDemo.git.

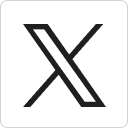



I will make sure to our Facebook page.