Warnings are not errors. Why do we bother to spend time fixing them? It feels so fast to get it compiled and run before looking into the warnings. It's because warnings may reveal that the code is not what you mean. It should be your habit to fix build warnings.
Errors or Warnings
Build errors are those that prevent you from getting a program or running it. Like it or not, you need to fix all the build errors. Warnings, on the other hand, don't have those restrictions. Anybody can ignore them and run the program. Luckily, the compiler usually has an option or switch to treat warnings as errors. By doing so, anybody has to address those warnings before they can continue to run the program. Having the compiler to treat warnings as errors is the only way to enforce everybody to fix the warnings too.
I'll show it how to do it in .Net Core and illustrate the importance of warnings by some examples.
Enable Warnings as Errors
There are two ways to enable warnings as errors.
The first one is to set the property TreatWarningsAsErrors
in the project file. for example, in the csproj file,
<PropertyGroup>
<TreatWarningsAsErrors>true</TreatWarningsAsErrors>
</PropertyGroup>
On the other hand, <NoWarn>
is used to exclude certain warnings
<NoWarn>$(NoWarn);CS0168;CS0219</NoWarn>
<WarningLevel>
is used to set the warning level. The higher the number is, the more less servere warnings it'll report. The default value is 4.
Second, use the compiler option -warnaserror
. For example,
dotnet build -warnaserror example.csproj
This will turn all warnings to errors. You can set specific warning with -warnaserror
like -warnaserror:642,649
. -nowarn
on the other hand, disables certain warnings.
-warn
is used to set the warning level.
The Warning Examples
warning CS4014: Because this call is not awaited, execution of the current method continues before the call is completed. Consider applying the 'await' operator to the result of the call.
This occurs when you forget to use
await
on anyasync
call. It reveals a mistake in the code even though it's not a build error. There may be a subtle bug when the program runs. It's not always happening which makes it much difficult to reproduce the bug and diagnose. For exampleusing (FileStream fs = File.Create(path)) { fs.WriteAsync(bytes, 0, byteSize, CancellationToken.None); }
Without
await
onfs.WriteAsync
, it's possible thatfs
is being disposed whileWriteAsync
is still running. It all depends on the scheduling. So it's quite difficult to diagnose.warning CS1717: Assignment made to same variable; did you mean to assign something else?
I'm sure you don't intend to assign to the same variable. It could be a typo. But you may not notice it in a quick glimpse. For example
By treating warnings as errors, you're forced to look at the message and look at what's going on.class Test { private int count; public Test(int count) { count = count; } }
Exceptions
No all warnings indicate mistakes. Spend some time thinking about that. If you can justify it, you can suppress the warnings. One way of doing that is to use <NoWarn>
in the project file or -nowarn
in the compiler option. I don't recommend it because it applies to the whole project. I suggest to use pragma warning to disable the warning. Remember to enable the warning afterwards. For example
#pragma warning disable CS3021
//code
#pragma warning restore CS3021
Take away
Always set the flag to treat warnings as errors in your build or compiler. Always fix warnings except you can justify not to. Always explicitly surpress certain warnings before the line and restore it afterward.

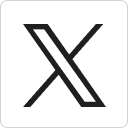


