Many languages have const and enum. The compiler treats enum values as constan integers too. Enum can be as bad as how const can be. In that sense, I'll use const as an example to demonstrate how they will go wrong.
The Good Side of a const
The meaning of const is, as it indicates, that the value is a constant. There are run time constants or compile time constants. The run time constant means that the value doesn't change when the program runs. The compile time constant indicates that the programmer shouldn't change the value of the variable. The compiler will forbid any assignments to the const except the first initialization. The are at the good side of a const when you don't want to change the value of the variable. It's encouraged in general. The compiler can also use the knowledge to optimize your code.
When It Goes Bad
It'll cause problems when you use a const in a shared library (.so) or dynamic library (.dll). Let me demonstrate it with an example in C++ on Linux. It'll be the same in C++ on other platforms or C# too.
1. Create a header file with a const in the class: ConstHeader.h
#ifndef __CONSTHEADER_H__
#define __CONSTHEADER_H__
const int TestConst = 10;
class ConstHeader
{
public:
ConstHeader();
int get_num() const;
private:
const int num;
};
#endif //__CONSTHEADER_H__
2. Create source file ConstHeader.cpp
#include "ConstHeader.h"
ConstHeader::ConstHeader() :
num(TestConst)
{
}
int ConstHeader::get_num() const
{
return num;
}
3. Create the program that uses the const: UseConst.cpp
#include
#include "ConstHeader.h"
using namespace std;
int main(int argc, char** argv)
{
ConstHeader header;
cout << "number in executable " << TestConst << endl
<< "number in library " << header.get_num() << endl;
return 0;
}
4. Compile ConstHeder.cpp into a shared library
$ g++ -shared -Wl,-soname,libConstHeader.so -o libConstHeader.so ConstHeader.cpp
5. Create the program linking to the shared library
$ g++ -o UseConst UseConst.cpp -L. -lConstHeader
6.Run the program
$ LD_LIBRARY_PATH=. ./UseConst
number in executable 10
number in library 10
That looks pretty good. The program uses the same const value as the one in the shared library.
Now what happen if we update the shared library?
Let's change the value of the const TestConst in the shared library
const int TestConst = 20;
Create the shared library and run the program again without recompiling
$ g++ -shared -Wl,-soname,libConstHeader.so -o libConstHeader.so ConstHeader.cpp
$ LD_LIBRARY_PATH=. ./UseConst
number in executable 10
number in library 20
Ooops. When it uses the const directly, it gets 10. While the shared library shows the value is 20.
What's Wrong
Let us pause a minute to think about what's changed. You use a const integer TestConst in a shared library. But the library is updated. Pay extra attentions to the const that are defined in a shared library. Sometimes, when the value is changed. it'll be pain in the ass to debug it. This is the same to an enum's implicit value. For example:
enum class Color
{
red = 0,
blue,
yellow,
};
If this is from a third party library and the enum Color
is changed in a new version. E.g.
enum class Color
{
red = 0,
green,
blue,
yellow,
};
Your program will be broken if you use Color::yellow
and Color::blue
and don't compile against to the updated header.
All the const and enums defined in the header files can be accessed from a separate compile unit. They are actually interfaces, part of the contract. As a library user, when you use an interface, you expect that the same interface doing the same things in all the versions of the library. Your application relies on that to function well. As a library author, you don't want to drive your user crazy. Don't change the public interfaces.
How to Mitigate It
It depends on your purpose. As a library author, if you just want to provide a well defined value to the library user, use a function to return the value. This will have some overhead in function call, but you can change the value in future version. In C#, you also can declare the variable readonly
instead of const
. Either way it won't become a compile time constant. Instead, the run time will read the value from your library and it still cannot be changed.
For enum, it'll be a little complicated. The first approach is that you always append the new enumerator at the end. Take Color
as an example, instead of adding green
in between red
and blue
, you always add the new enumerator after the last one: yellow
. A second approach is that you always set explicit value to the enumerator as what we do to red
. There are problems in collaborative work in a large team. There is no way to enforce a person to append to the last in the first approach. For the second approach, two people may use the same explicit value for new enumerators in their work, and they all check in at the same time. Comments in the code. That won't always help.
Remember, public consts and enums are interfaces. Don't change them. This is the best option to prevent them going bad.

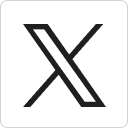


